
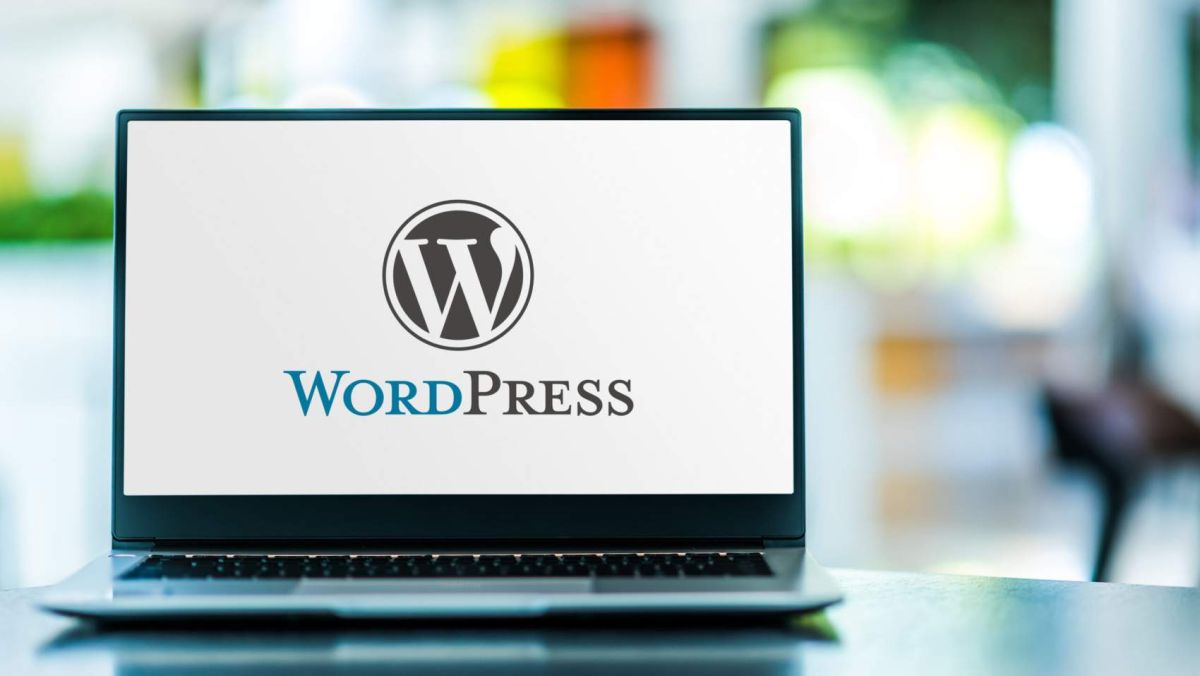
Handlebars.js is a simple templating language that enables dynamic content rendering within HTML. When combined with WordPress, Handlebars.js can streamline the creation of dynamic templates, making it easier to separate the logic and presentation in your themes or plugins. In this guide, we’ll cover how to use Handlebars.js effectively within a WordPress environment to enhance your site’s flexibility.
Prerequisites
- Familiarity with WordPress themes or plugins development.
- Basic knowledge of JavaScript and PHP.
- A local or remote WordPress installation for testing purposes.
- An understanding of WordPress’ theme structure and hooks.
Step-by-Step Guide
Step 1: Install and Enqueue Handlebars.js in WordPress
Before using Handlebars.js in WordPress, you need to include it in your theme or plugin. Here’s how you can do that:
- Download Handlebars.js
You can download Handlebars.js from the official website or use a CDN link to include it. - Enqueue Handlebars.js in Your WordPress Theme/Plugin
Open your theme’s
functions.php
file or the main plugin file and add the following code to enqueue Handlebars.js:function enqueue_handlebars() { wp_enqueue_script('handlebars', 'https://cdn.jsdelivr.net/npm/handlebars@latest/dist/handlebars.min.js', array(), null, true); } add_action('wp_enqueue_scripts', 'enqueue_handlebars');
This will ensure that Handlebars is loaded on every page where it’s needed.
Step 2: Set Up a Basic Template
Once you’ve included Handlebars.js, you can start creating templates. Let’s create a simple Handlebars template in your WordPress theme.
- Create an HTML Template
In one of your theme files (such as
single.php
orpage.php
), add a script tag for your Handlebars template:<script id="post-template" type="text/x-handlebars-template"> <div class="post-item"> <h2>{{title}}</h2> <p>{{content}}</p> <p><small>Posted on: {{date}}</small></p> </div> </script>
This template will render a post with the title, content, and date.
- Create a Container for Rendering
Below the template, add a container where you want the rendered content to appear:
<div id="posts-container"></div>
Step 3: Fetch Data from WordPress Using PHP
You can use PHP to pass dynamic data from WordPress to JavaScript for rendering in Handlebars templates.
- Pass Data from PHP to JavaScript
Add a PHP script that fetches data from the WordPress database and makes it available in the frontend using
wp_localize_script()
.function localize_posts_data() { $posts_data = array(); $query = new WP_Query(array( 'posts_per_page' => 5, 'post_status' => 'publish', )); if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); $posts_data[] = array( 'title' => get_the_title(), 'content' => get_the_excerpt(), 'date' => get_the_date(), ); } } wp_localize_script('handlebars-custom', 'postsData', array( 'posts' => $posts_data )); wp_enqueue_script('handlebars-custom', get_template_directory_uri() . '/js/handlebars-custom.js', array('handlebars'), null, true); } add_action('wp_enqueue_scripts', 'localize_posts_data');
This code retrieves the latest 5 published posts and passes them to the
postsData
JavaScript object, which you can now access in the frontend.
Step 4: Render the Data with Handlebars.js
Now that you’ve set up the template and passed data to JavaScript, let’s use Handlebars.js to render it.
- Create a Custom JavaScript File
In your theme or plugin folder, create a file named
handlebars-custom.js
and add the following code:document.addEventListener("DOMContentLoaded", function () { // Get the template from the HTML var source = document.getElementById('post-template').innerHTML; // Compile the template with Handlebars var template = Handlebars.compile(source); // Loop through the data passed from PHP and render it postsData.posts.forEach(function(post) { var html = template(post); document.getElementById('posts-container').innerHTML += html; }); });
This code listens for the
DOMContentLoaded
event, compiles the Handlebars template, and then loops through the data passed from PHP. Each post is rendered and appended to theposts-container
div.
Step 5: Customize and Extend Handlebars Functionality
Once you’ve set up the basic rendering, you can start adding more advanced Handlebars features such as helpers and partials to customize your templates further.
- Using Handlebars Helpers
Helpers allow you to run JavaScript logic inside your templates. For example, you can create a helper to truncate long post titles:
Handlebars.registerHelper('truncate', function (str, len) { if (str.length > len) { return str.substring(0, len) + '...'; } return str; });
You can then use this helper in your Handlebars template:
<h2>{{truncate title 20}}</h2>
- Using Handlebars Partials
Partials allow you to break your templates into reusable pieces. For example, you can create a partial for rendering the post date:
In your JavaScript:
Handlebars.registerPartial('postDate', '<p><small>Posted on: {{date}}</small></p>');
In your template:
<div class="post-item"> <h2>{{title}}</h2> <p>{{content}}</p> {{> postDate}} </div>
Step 6: Debugging and Optimization
To ensure smooth performance and debugging:
- Use the Browser’s Developer Tools
Use the console to ensure that data is being passed correctly to JavaScript and that templates are rendering as expected.
- Minify JavaScript Files
To improve load times, make sure to minify your
handlebars-custom.js
file in a production environment. You can use tools like UglifyJS. - Cache Handlebars Templates
If your templates don’t change frequently, consider caching them to improve performance, especially on larger websites. You can store the compiled templates in local storage or server-side caching mechanisms.
Conclusion
By integrating Handlebars.js with WordPress, you can significantly improve the flexibility and maintainability of your WordPress themes or plugins. You now have the knowledge to set up Handlebars.js in WordPress, pass dynamic data using PHP, and render content dynamically with Handlebars templates. As you grow more comfortable with this setup, you can explore more advanced Handlebars.js features, such as custom helpers and partials, to create reusable, dynamic components within your WordPress site.
